To restrict user creation to administrators only, you can utilize Cognito. You can achieve this by using the AdminCreateUser
function in the Amazon Cognito user pool API.
To implement the API, you can utilize the Serverless Framework
.
What you want to make
When the administrator includes the email address of the person requesting the account in the request and proceeds with the execution, the account is created. You need to create an API that can send the username and initial password to the specified email address.
Cognito user pool settings
This time, we will proceed with the implementation when using only the email address.
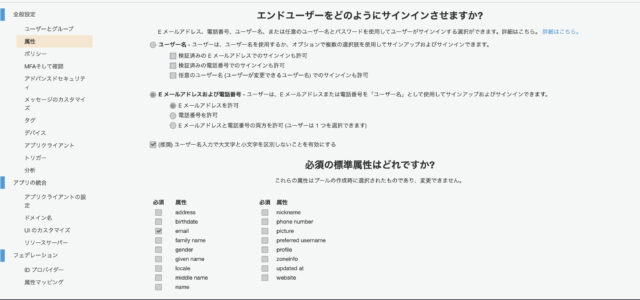
“Do you want to allow users to self-sign up?” you must specify “Allow administrators to create users only“.
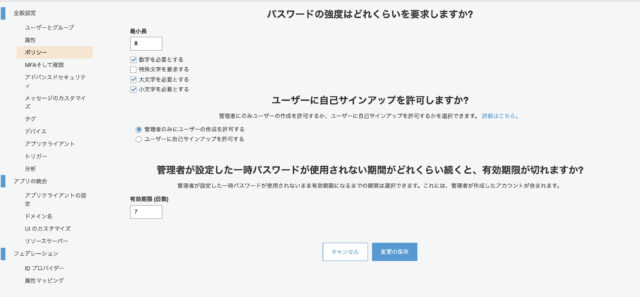
Folder configuration
- .serverless
- ...
- conf
- ...
- functions
- auth
- index.js
- adminCreateUser.js
- node_modules
- ...
- package-lock.json
- package.json
- serverless.yml
- webpack.config.js
implementation
app: app-name
service: service-name
provider:
name: aws
runtime: nodejs12.x
region: ap-northeast-1
stage: ${opt:stage, self:custom.defaultStage}
profile: default
environment:
${file(./conf/${opt:stage}/${opt:stage}.yml)}
iamRoleStatements:
- Effect: Allow
Action:
- cognito-idp:*
Resource:
- "arn:aws:cognito-idp:ap-northeast-1:**********:userpool/ap-northeast-1_******"
custom:
defaultStage: dev
otherfile:
environment:
dev: ${file(./conf/dev/dev.yml)}
stg: ${file(./conf/stg/stg.yml)}
prd: ${file(./conf/prd/prd.yml)}
functions:
adminCreateUser:
handler: functions/auth/index.adminCreateUser
events:
- http:
path: owner/create
method: post
cors: true
plugins:
- serverless-webpack
const validator = require('validator')
const qs = require('qs')
const cognito = require('./adminCreateUser.js')
export function adminCreateUser(event, context, callback) {
var pParam = null
if(event.body !== null) {
pParam = (validator.isJSON(event.body)) ? JSON.parse(event.body) : qs.parse(event.body)
}
cognito.adminCreateUser(pParam, (err, result) => {
if (err) {
callback(null, {
statusCode: 400,
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers': 'Content-Type'
},
body: JSON.stringify({
status: '400',
errorInfo: err
})
})
} else {
callback(null, {
statusCode: 200,
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers': 'Content-Type'
},
body: JSON.stringify({
status: '000',
responseData: result
})
})
}
})
}
'use strict'
const AWS = require('aws-sdk')
exports.adminCreateUser = (body, callback) => {
if (body.email === undefined) return callback('email is a required field.) ')
var cognitoisp = new AWS. CognitoIdentityServiceProvider({ apiVersion: '2016-04-18' })
var params = {
UserPoolId: 'ap-northeast-1_*****',
Username: body.email,
DesiredDeliveryM[ 'EMAIL' ]ediums: ,
ForceAliasCreation: false,
UserAttributes: [
{
Name: 'email_verified',
Value: 'true'
},
{
Name: 'email',
Value: body.email
}
]
}
cognitoisp.adminCreateUser(params, function(err, data) {
if (err) {
console.error(err, err.stack)
callback(err)
} else {
console.log(data)
callback(null, data)
}
})
}
Reference
For the time being, look at this ↓
Check the implementation contents ↓
↑ In the implementation, because the mail did not fly ↓