Cognitoで「管理者のみにユーザーの作成を許可する」とした場合の実装を行う。Amazon CognitoユーザープールAPIのAdminCreateUser
を使用する。
APIの実装には、Serverless Flamework
を使用する。
つくりたいもの
管理者がアカウントを発行したい人のメールアドレス
を投げると、アカウントが発行され、そのメールアドレス宛にユーザ名と初期パスワード
が送信されるAPI
Cognitoユーザープールの設定
今回は、メールアドレスのみを利用する場合で実装を進めます。
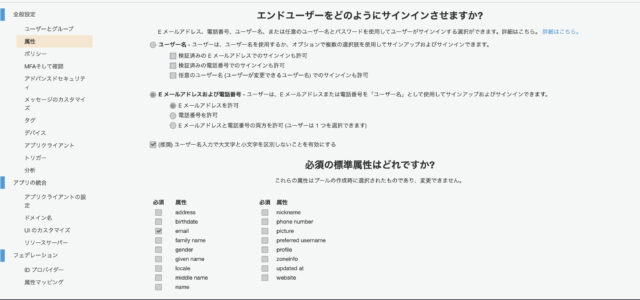
「ユーザに自己サインアップを許可しますか?」に対して、「管理者のみにユーザの作成を許可する」を指定する必要があります。
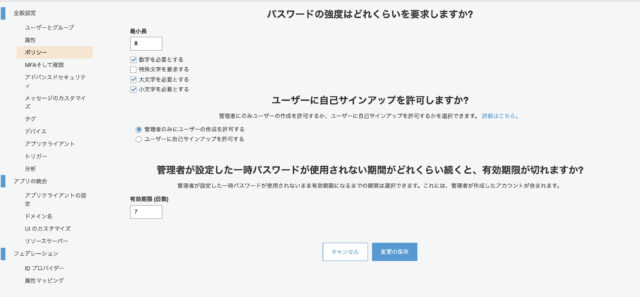
フォルダ構成
- .serverless
- ...
- conf
- ...
- functions
- auth
- index.js
- adminCreateUser.js
- node_modules
- ...
- package-lock.json
- package.json
- serverless.yml
- webpack.config.js
実装
app: app-name
service: service-name
provider:
name: aws
runtime: nodejs12.x
region: ap-northeast-1
stage: ${opt:stage, self:custom.defaultStage}
profile: default
environment:
${file(./conf/${opt:stage}/${opt:stage}.yml)}
iamRoleStatements:
- Effect: Allow
Action:
- cognito-idp:*
Resource:
- "arn:aws:cognito-idp:ap-northeast-1:**********:userpool/ap-northeast-1_******"
custom:
defaultStage: dev
otherfile:
environment:
dev: ${file(./conf/dev/dev.yml)}
stg: ${file(./conf/stg/stg.yml)}
prd: ${file(./conf/prd/prd.yml)}
functions:
adminCreateUser:
handler: functions/auth/index.adminCreateUser
events:
- http:
path: owner/create
method: post
cors: true
plugins:
- serverless-webpack
const validator = require('validator')
const qs = require('qs')
const cognito = require('./adminCreateUser.js')
export function adminCreateUser(event, context, callback) {
var pParam = null
if(event.body !== null) {
pParam = (validator.isJSON(event.body)) ? JSON.parse(event.body) : qs.parse(event.body)
}
cognito.adminCreateUser(pParam, (err, result) => {
if (err) {
callback(null, {
statusCode: 400,
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers': 'Content-Type'
},
body: JSON.stringify({
status: '400',
errorInfo: err
})
})
} else {
callback(null, {
statusCode: 200,
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers': 'Content-Type'
},
body: JSON.stringify({
status: '000',
responseData: result
})
})
}
})
}
'use strict'
const AWS = require('aws-sdk')
exports.adminCreateUser = (body, callback) => {
if (body.email === undefined) return callback('emailは必須項目です。')
var cognitoisp = new AWS.CognitoIdentityServiceProvider({ apiVersion: '2016-04-18' })
var params = {
UserPoolId: 'ap-northeast-1_*****',
Username: body.email,
DesiredDeliveryMediums: [ 'EMAIL' ],
ForceAliasCreation: false,
UserAttributes: [
{
Name: 'email_verified',
Value: 'true'
},
{
Name: 'email',
Value: body.email
}
]
}
cognitoisp.adminCreateUser(params, function(err, data) {
if (err) {
console.error(err, err.stack)
callback(err)
} else {
console.log(data)
callback(null, data)
}
})
}
参考
とりあえず、見るとこ↓
AdminCreateUser - Amazon Cognito User Pools
Creates a new user in the specified user pool.
実装内容の確認して↓
Just a moment...
↑の実装では、メールが飛ばなかったので↓
Just a moment...