There are several ways to determine that an object is empty (={}
) in javaScript, so make a note of it.
Object.keys(obj).length
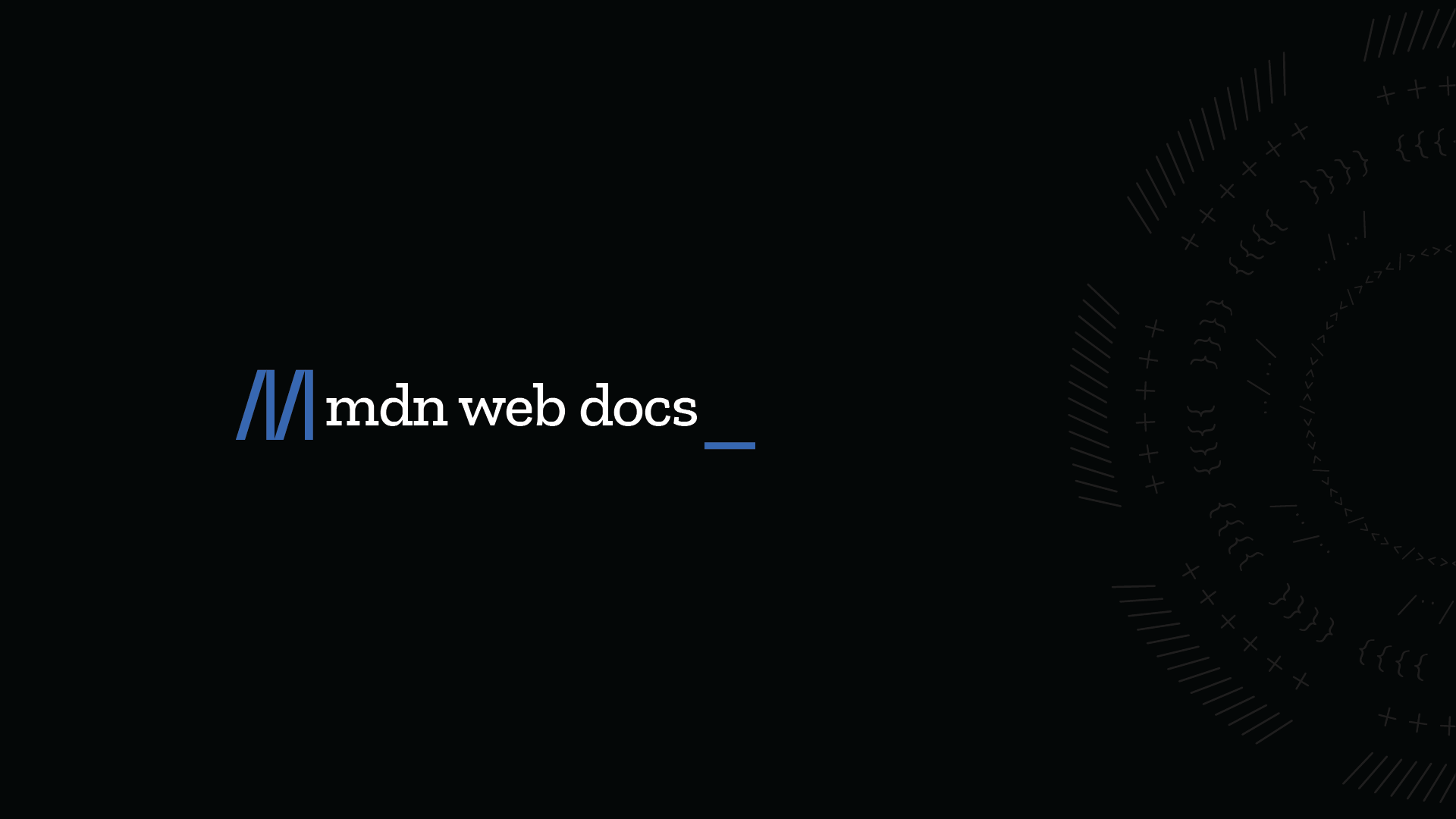
Here are some of the many things I’ve researched.
This is how to get and determine the Key array of Objects using Object.keys(obj).length
.
function isEmpty(obj) {
return ! Object.keys(obj).length
}
console.log(isEmpty({})) // -> true
console.log(isEmpty({'a': 'a'})) // -> false
You can use the same Object.keys(obj).length
to judge even if it looks like this.
var obj = {}
console.log(0 === Object.keys(obj).length) // -> true
console.log(! Object.keys(obj).length) // -> true
for(… in…)
The above-mentioned method of using Object.keys().length
seems to be extremely slow depending on the chrome version.
The current one seems to have already been fixed, but if you have to deal with an older version, you may need to consider using the following:
function isEmpty(obj) {
for (let i in obj) {
return false
}
return true
}
console.log(isEmpty({})) // -> true
console.log(isEmpty({'a': 'a'})) // -> false
As you can guess from here, you may see slowing down in other browsers (older versions of ).
JSON.stringify(obj)
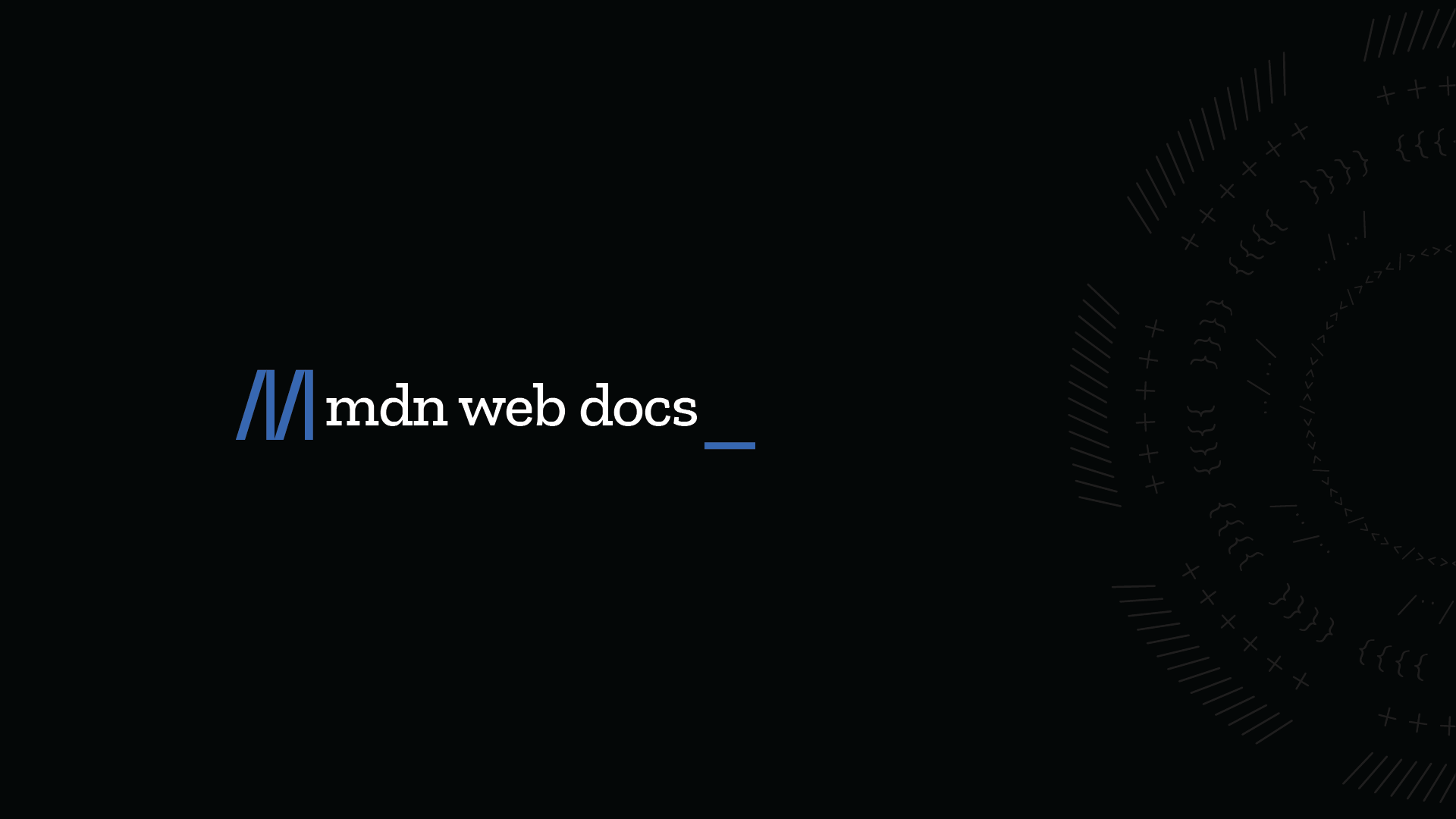
I can’t do this, but it seems to be slow. The details are unknown because we have not measured it.
var obj = {}
console.log(JSON.stringify(obj) === '{}') // -> true
Summary
Basically, if you use the first Object.keys(obj).length
, it seems to be no problem.
If you need to make this decision over and over again, you need to check the operating speed and choose what to use.